Angular is a typescript-based web application framework and is Google’s brilliant creation. It has released its latest version which is Angular 14. It has arrived with stand-alone components, promising to streamline application development by reducing the need for angular modules.
It is believed to be the most systematic pre-planned upgrade by Angular. It has released new features which include CLI auto-completion, typed reactive forms, stand-alone components, directives, pipes, and enhanced template diagnostics.
Stand-alone Components:
The standalone component is a new feature that lets you create your components and use them anywhere in the app. The biggest advantage of standalone components is that they are easier to customize than other types of components.
You can create these kinds of components using the @Component annotation, which tells angular how to build this kind of component when you include it in the app. Here’s an example:
[code language=”css”]
import {Component} from ‘@angular/core’;
import {AppComponent} from ‘./app.component’;
@Component({ selector: demo-app’, template: `<h1>Hello World!</h1>`}) export class AppComponent {}
[/code]
Strictly Typed Forms:
In Angular 14, you can now use TypeScript to enforce a strict form of types on the forms. This means that each field will be checked against its type when it is submitted or validated, which makes it easier to make sure that the forms are not invalid.
The strictly typed forms have been improved as follows:
- More responsive look and feel
- The form is now easier to use and less confusing to the users
- Form validation errors are displayed at once on the screen instead of having them displayed as pop-ups after submitting data
Never miss an update from us. Join 10,000+ marketers and leaders.
Angular CLI Auto-Completion
Angular CLI auto-completion is a new feature that provides auto-completion for the angular command line interface (CLI) commands. The completion feature is based on the TypeScript definition files and uses the IntelliJ IDEA plugin.
It can be enabled by adding @types/angular to the list of types you want to use in the app. In addition, you can select which kinds of completion you want such as TypeScript or ECMAScript features related to angular.
The auto-completion feature in the Angular CLI gives the ability to type < and then press Tab or Shift+Tab to complete the selection. You can also use Ctrl+Space or Command+Space to make the selections by typing words in the console.
In addition, it will turn on the tab completion automatically when you are in an editor window or running an application. This makes it easier for developers who don’t have a lot of experience with Angular to get started with it quickly.
Improved Template Diagnostics
The ng template debug method is removed from the new release of the framework. To ensure that you have time to migrate the new way of debugging template syntax errors in Angular 14. We are introducing a new diagnostic method called ng-template-error. This diagnostic will print the offending code within an error message when the app throws an error during the runtime.
It can enable this new diagnostic by including the following @Output decorator on all of the components:
[code language=”css”]
import { NgModule } from ‘@angular/core’;
import { BrowserModule } from ‘@angular/platform browser;
import { DemoApp } from `./app`; import { AppComponent } from `./app.component`;
@NgModule({ imports: [ BrowserModule ], declarations: [ AppComponent ], bootstrap: [ AppComponent ] }) export class AppModule {}
[/code]
Streamlined Page Title Accessibility
The page title is the most visible piece of information on a web page. It is what users see when they open a new tab or window and it is what search engines used to index the content.
You can customize the title tag of your pages directly from within the <head> of your style sheet. You can now leverage the power of the HTML to provide additional context for the title tag.
In the previous release, Angular had to assume that the title property of
elements was accessible. However, this assumption was not always accurate and could lead to unexpected behavior.
Now, Angular can assume that the title property is accessible when it needs to be. This means that you will see fewer exceptions when using
elements with a title attribute in the application.
You can also opt-in to using this new feature by configuring the app with @angular/platform-server@2.0.0-beta.18 or higher and adding the following element in the component’s template:
[code language=”css”]
<%= raw `<ng-content></ng-content>` %>
[/code]
In Angular 14, the page title is accessible via a simple API. If you have a component with a template and a pipe that returns an object, you can use the title property on the returned object to access the page title.
[code language=”css”]
import { Component } from ‘@angular/core’;
import { RouterLink } from ‘@angular/router’;
import { NavController } from ‘@angular/common;
import { AppComponent } from ‘./app.component’;
@Component({ selector: ‘app-root’, styleUrls: [‘./app.component.css’], template: `<router-outlet></router-outlet>; ` }) export class AppComponent {}
export function getTitle(): any;
export const router = new RouterLink({ path: ‘/’, title: getTitle() });
[/code]
Latest Primitives in the Angular CDK
The Angular CDK (Compiler-Dependent JavaScript) is a library that provides a set of primitives for building components, services, and many other types of applications.
The latest version of the CDK is now available in an alpha release with several new features:
- Angular Elements: A new way to build HTML elements, which can be placed inside the other components or used standalone as an <div> element. The new elements are inspired by the ShadowDOM APIs in V8 and Web Components.
- New FormBuilder: An Angular form builder that allows you to create forms with ease using simple declarative expressions and properties instead of creating a separate controller for each form field.
There are a bunch of new primitives in the Angular CDK, including:
- @Output () decorator: It allows you to write template code directly into your component class.
- @Injectable () decorator: This allows you to inject any other component or service into your component class.
- @Link () decorator: This allows you to create link tags that can be used in templates and directives.
I’ve worked with the team at Andolasoft on multiple websites. They are professional, responsive, & easy to work with. I’ve had great experiences & would recommend their services to anyone.
Ruthie Miller, Sr. Mktg. Specialist
Salesforce, Houston, Texas
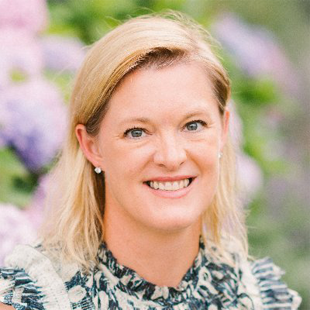
Conclusion
Angular 14 is finally released with some amazing new features and updates. This version will have a major impact on the development community as a whole.
The Angular developer community strives to make sure that web developers get better versions of the framework allowing them to stay updated with the rest of the online ecosystem and users’ needs.