Ruby on Rails aka “RoR” is an open-source MVC framework built using the Ruby programming language.
It is considered as the epitome of the latest generation of high-productivity, open source web development tool. The growing demand for Ruby on Rails has been driven by successful RoR development companies like Andolasoft, who benefit from the speed and agility of building applications in Rails, which results in increased productivity and company growth.
1. Framework Built on Agile Methodology
RoR is faster because the framework was built based on Agile development methodology, best practices are emulated so web development is done with high quality and speed.
RoR framework includes support programs, compilers, code libraries, tool sets, and application programming interfaces (APIs) that bring together the different components to enable development of a project or solution.
Never miss an update from us. Join 10,000+ marketers and leaders.
It’s possible to build sites that used to take 12 weeks in just 6 weeks using Ruby on Rails. It means you can save 50% on your development expenses.
2. Less Code Writing
Rails creates a skeleton for an application or module by executing all the code libraries. You must write some commands to accomplish this. This is what makes the process of web development faster.
Rails makes database deployments simpler than any open, or proprietary solution by allowing for migrations.
-
Adopting principle of DRY
It also adopts the principle of “Don’t Repeat Yourself”. So all information can be retrieved from a single unambiguous database which tends to easier development.
-
Easy Configuration
A key principle of Ruby on Rails development is convention over configuration. This means that the programmer does not have to spend a lot of time configuring files in order to get setup, Rails comes with a set of conventions which help speeding up development.
-
Modular Design
Ruby code is very readable and mostly self-documenting. This increases productivity, as there is little need to write out separate documentation, making it easier for other developers to pick up existing projects.
-
Designer Friendly Templates
HTML templates is a core feature of Rails. It allow templates to be composed of a base template that defines the overall layout of the page,the base template is called a “layout” while the individual page templates are “views”. The Rails application retrieves the list of tags from the database and makes it available to the view.The section of the view that renders these category is:
<%= render :partial => 'category' %>
This refers to a Ruby partial, a fragment of a page, which is contained in _category.html.erb. That file’s content is:
<h3>categories</h3> <p class="categorylist"> <%= render :partial => 'categorylist', :collection => @category %> </p>
- This generates the heading then refers to another partial, which will be used once for each object in the collection named “categorylist”.
3. Third Party Plugin/Gem Support
Mature plugin architecture, well used by the growing community. Rails plugins allows developer to extend or override nearly any part of the Rails framework, and share these modifications with others in an encapsulated and reusable manner.
Rails community provides a wealth of plugins as Ruby Gems that you simply add to your project Gem-file and install. This significantly accelerates development and maintenance time as you’re not trying to integrate disparate libraries, it’s already done for you.
4. Automated Testing
Rails has developed a strong focus on testing, and has good testing suit in-built within the frameworks.
Rails makes it super easy to write your tests. It starts by producing skeleton test code while you are creating your models and controllers.
I’ve worked with the team at Andolasoft on multiple websites. They are professional, responsive, & easy to work with. I’ve had great experiences & would recommend their services to anyone.
Ruthie Miller, Sr. Mktg. Specialist
Salesforce, Houston, Texas
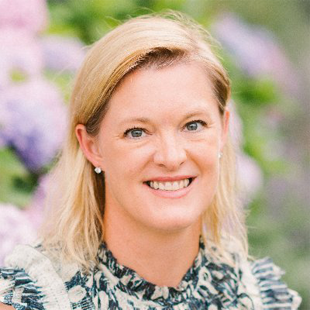
Rails ships with a built-in test suite. There are many tools available in the market for testing Rails application as mentioned below with other web apps from many different aspects.
- Rspec
- Cucumber
- Test Unit
- Shoulda
- Selenium (not really a ruby thing but more of a web thing)
But if you are new to testing we highly recommend you start with learning Rails own testing suite before start using other tools
5. Easier Maintenance
Once site launches, future modifications to your site (e.g., adding new features, making changes to the data model) can be made more quickly, for the same reasons noted above.
New features can be added quickly and maintaining applications over time can also be more cost-effective.
If you like this one, you might also like Why Rails framework popular among Ruby developers and The Best practices for Rails App Development .